Create a MOC from an astropy Table¶
[1]:
import matplotlib.pyplot as plt
from astropy import units as u
from astropy.visualization.wcsaxes.frame import EllipticalFrame
from astropy.wcs import WCS
from astroquery.vizier import Vizier
from mocpy import MOC
Let’s load a VizieR catalog
[2]:
viz = Vizier(columns=["*", "_RAJ2000", "_DEJ2000"])
viz.ROW_LIMIT = -1 # sets the limit to infinity
table = viz.get_catalogs("I/293/npm2cros")[0]
print(table)
_RAJ2000 _DEJ2000 NPM2 KLA RAJ2000 ... IQ IN r_Star mag tyc
deg deg ... mag
---------- ---------- -------- --- ---------- ... --- --- ------ ----- ---
339.638333 83.166667 +83.0016 HPM 22 38 33.2 ... 0 0 811 14.20
339.638333 83.166667 +83.0016 HPM 22 38 33.2 ... 0 0 813 14.20
349.530833 83.010556 +83.0022 VAR 23 18 07.4 ... 0 0 843 -- T
349.530833 83.010556 +83.0022 SRA 23 18 07.4 ... 0 0 1 9.60 T
323.854167 82.997222 +82.0030 UBV 21 35 25.0 ... 0 0 5 10.08 T
332.107917 82.747500 +82.0055 WD 22 08 25.9 ... 0 0 1012 16.00
332.933750 82.607778 +82.0059 HPM 22 11 44.1 ... 0 0 811 16.70
335.088750 82.970833 +82.0065 HPM 22 20 21.3 ... 0 0 811 11.00 T
337.119167 82.763889 +82.0074 UBV 22 28 28.6 ... 0 0 5 10.42 T
337.274583 82.662222 +82.0075 HPM 22 29 05.9 ... 1 0 811 17.40
... ... ... ... ... ... ... ... ... ... ...
257.227500 -23.094167 -23.0151 ELS 17 08 54.6 ... 0 0 511 13.50
267.989583 -23.012222 -23.0162 CMC 17 51 57.5 ... 0 0 817 11.13 T
267.989583 -23.012222 -23.0162 ELS 17 51 57.5 ... 0 0 252 -- T
270.244583 -23.032222 -23.0164 CMC 18 00 58.7 ... 0 0 817 10.52 T
270.244583 -23.032222 -23.0164 EA 18 00 58.7 ... 0 0 1 10.20 T
270.337500 -23.000556 -23.0165 COM 18 01 21.0 ... 0 0 819 11.20 T
270.442917 -23.020000 -23.0166 UNK 18 01 46.3 ... 0 0 816 14.00
270.474167 -23.028889 -23.0167 COM 18 01 53.8 ... 1 0 819 11.60 T
271.357083 -23.005556 -23.0168 ELS 18 05 25.7 ... 0 0 252 -- T
271.357083 -23.005556 -23.0168 OB 18 05 25.7 ... 0 0 393 9.70 T
Length = 46887 rows
Let’s create a MOC from the coordinates of this table
[3]:
moc = MOC.from_lonlat(
table["_RAJ2000"].T * u.deg,
table["_DEJ2000"].T * u.deg,
max_norder=6,
)
[4]:
fig = plt.figure(figsize=(9, 5))
galactic_wcs = WCS(
{
"naxis": 2,
"naxis1": 1620,
"naxis2": 810,
"crpix1": 810.5,
"crpix2": 405.5,
"cdelt1": -0.2,
"cdelt2": 0.2,
"ctype1": "GLON-AIT",
"ctype2": "GLAT-AIT",
},
)
ax = fig.add_subplot(1, 1, 1, projection=galactic_wcs, frame_class=EllipticalFrame)
moc.fill(
ax=ax,
wcs=galactic_wcs,
edgecolor="teal",
facecolor="orange",
linewidth=1.0,
fill=True,
alpha=0.8,
)
moc.border(ax=ax, wcs=galactic_wcs, color="k")
ax.grid(visible=True)
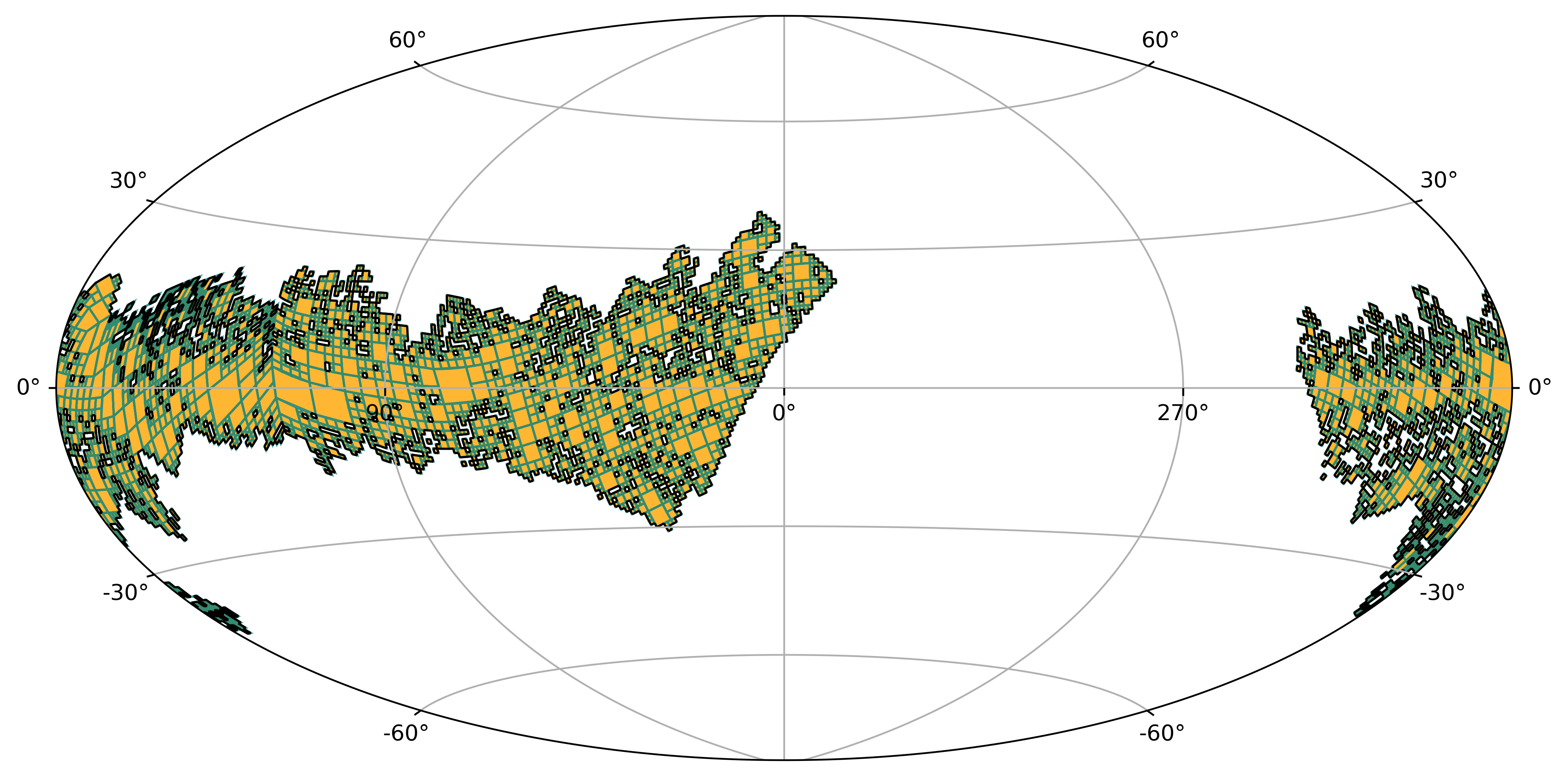