API¶
This is the API for the cdshealpix Python package.
This package is a wrapper around the Rust cdshealpix crate. The functions currently implemented by cdshealpix are the following:
cdshealpix¶
The HEALPix cells can be represented following two schema. The nested and the ring one.
Here are two methods in the cdshealpix
base module allowing to
convert HEALPix cells represented in the nested scheme into cells
represented in the ring scheme and vice versa.
|
Convert HEALPix cells from the NESTED to the RING scheme. |
|
Convert HEALPix cells from the RING to the NESTED scheme. |
cdshealpix.nested¶
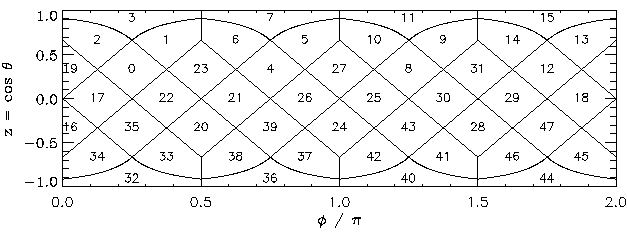
The HEALPix cells at \(depth=1\) represented in the nested scheme¶
|
Get the HEALPix indexes that contains specific sky coordinates. |
|
Get the HEALPix indexes that contains specific sky coordinates. |
|
Get the longitudes and latitudes of the center of some HEALPix cells. |
|
Get the coordinates of the center of a healpix cell. |
|
Project the center of a HEALPix cell to the xy-HEALPix plane. |
|
Project sky coordinates to the HEALPix space. |
|
Project coordinates from the HEALPix space to the sky coordinate space. |
|
Get the longitudes and latitudes of the vertices of some HEALPix cells at a given depth. |
|
Get the sky coordinates of the vertices of some HEALPix cells at a given depth. |
|
Get the neighbouring cells of some HEALPix cells at a given depth. |
|
Get the neighbours of specific healpix cells. |
|
Get the HEALPix cells contained in a cone at a given depth. |
|
Get the HEALPix cells contained in a polygon at a given depth. |
|
Get the HEALPix cells contained in an elliptical cone at a given depth. |
|
Compute the HEALPix bilinear interpolation from sky coordinates. |
cdshealpix.ring¶
The ring scheme HEALPix methods take a nside
parameter instead
of a depth
one.
nside
refers to the number of cells being contained in the side
of a base cell (i.e. the 12 cells at the depth
0).
While in the nested scheme, nside
is a power of two
(because \(N_{side} = 2 ^ {depth}\)), in the ring scheme,
nside
does not necessary have to be a power of two!
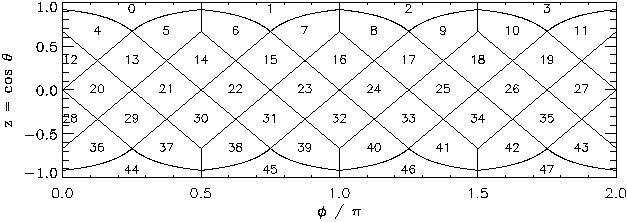
The HEALPix cells at \(N_{side}=2\) represented in the ring scheme¶
|
Get the HEALPix indexes that contains specific sky coordinates. |
|
Get the HEALPix indexes that contains specific sky coordinates. |
|
Get the longitudes and latitudes of the center of some HEALPix cells at a given depth. |
|
Get the sky coordinates of the center of some HEALPix cells at a given nside. |
|
Project the center of a HEALPix cell to the xy-HEALPix plane. |
|
Get the longitudes and latitudes of the vertices of some HEALPix cells at a given nside. |
|
Get the sky coordinates of the vertices of some HEALPix cells at a given nside. |
cdshealpix.skymap¶
This module provides a minimal interface to interact with Skymaps, as defined in the data format for gamma ray astronomy specification.
- class cdshealpix.skymap.Skymap(values)¶
A Skymap, containing values to associate to healpix cells.
- property depth¶
The depth of the skymap.
Avoids the costly log calculation.
- Returns:
int
The depth of the skymap.
Examples
>>> from cdshealpix.skymap import Skymap >>> map = Skymap.from_array([0]*12) >>> map.depth 0
- classmethod from_array(values)¶
Create a skymap from an array.
- Parameters:
- values
numpy.array
An array-like object. It should be one-dimensional, and its length should be the number of cells in a HEALPix order. It should be in the nested ordering (not tested).
- values
- Returns:
Skymap
A skymap object.
Examples
>>> from cdshealpix.skymap import Skymap >>> import numpy as np >>> skymap =Skymap.from_array(np.array([0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11], dtype=np.uint8))
- classmethod from_fits(path: str | Path)¶
Read a skymap in the nested schema from a FITS file.
This reader supports files which are:
all sky maps
in the nested scheme
and the implicit format
- Parameters:
- pathstr,
pathlib.Path
The file’s path.
- pathstr,
- Returns:
Skymap
A skymap. Its values are in a numpy array which data type in inferred from the FITS header.
- quick_plot(*, size=256, convert_to_gal=True, path=None)¶
Preview a skymap in the Mollweide projection.
- Parameters:
- size
int
, optional The size of the plot in the y-axis in pixels. It fixes the resolution of the image. By default 256
- convert_to_gal
bool
, optional Should the image be converted into a galactic frame? by default True
- path
str
orpathlib.Path
, optional If different from none, the image will not only be displayed, but also saved at the given location. By default None
- size
- to_fits(path)¶
Write a Skymap in a fits file.
- Parameters:
- path
str
,pathlib.Path
The file’s path.
- path